Console

개발할 때 가장 많이 사용하는 것이 바로 console.log()이다. 백엔드에서 새롭게 받은 request의 결과값을 확인한다거나 내가 짠 함수의 동작을 확인한다거나 등등 많은 동작에 사용된다. 아마 나뿐만 아니라 많은 웹개발자들이 사용중일 것이다.
오늘은 이렇게 자주 사용하는 console!
Node.js에서의 이 console에 대해서 알아보자.
console.log
가장 잘알려진 console.log이다. 주어진 인자를 출력한다.
console.log('🍎');
// 출력: 🍎
console.log(1 + 2);
// 출력: 3
console.log(1,2,3);
// 출력: 1,2,3
위의 console.log(1,2,3) 처럼 여러 인자를 받아 출력할수도 있다.
console.info
info를 보면 알수있듯이 말그대로 정보를 출력해준다. console.log와 같은 기능을 한다.
console.info('Information');
// 출력: Information
console.error
개발 중 에러가 난 경우 아래와 같이 빨간색의 문구를 콘솔창에서 자주 봤을 것이다.

위는 'Img Error!'라는 에러를 console.error()로 출력한 결과이다. 에러 메세지와 에러가 발생한 코드의 라인 번호나 에러가 난 코드 등 에러에 관한 정보를 담고 있다.
console.warn
개발 중 노란색의 warning 문구도 자주 보게된다.
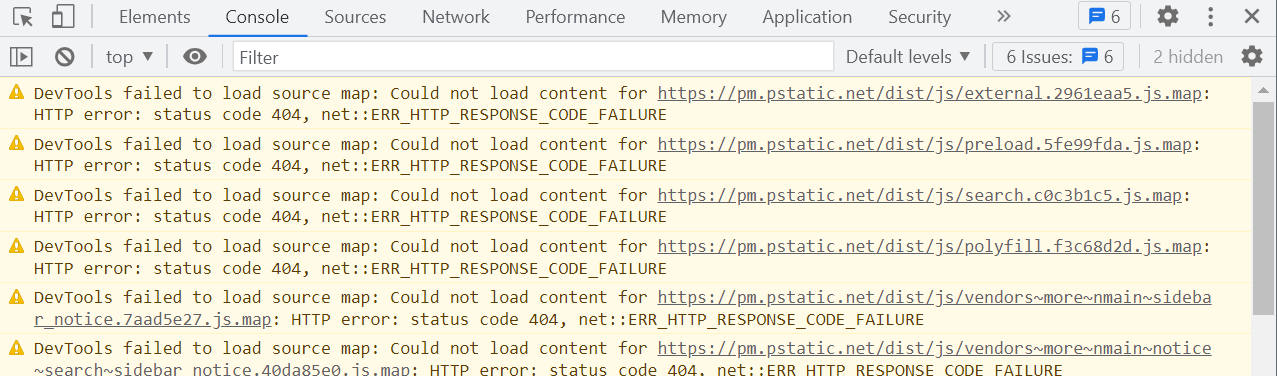
이러한 노란색 경보 문구로 경보를 표시하고 싶다면
console.warn('Warning!');
// 출력: Warning!
console.warn을 사용하면 된다.
console.assert
// console.assert(판별값, 출력할 메세지);
console.assert(true, 'True!');
console.assert(false, 'False!');
// 출력: False!
console.assert(1 < 2, 'Nope');
// 출력: Nope
assert에 true인 인자가 주어지면 출력되지 않는다. 그와 반대로 false값인 인자가 주어지면 메세지가 출력된다.
console.dir
객체를 출력할때 사용한다.
let company = {
name: "ABC corp",
industry: "Fashion",
detail: { Boss: "Jake", Address: "Seoul" },
};
console.dir(company);
// 출력: { name: 'ABC corp', industry: 'Fashion', detail: { Boss: "Jake", Address: "Seoul" }}
console.dir(company, { showHidden: true, colors: false, depth: 2 });
// depth가 2이므로 detail의 전체 value가 출력된다.
// 출력: {
name: 'ABC corp',
industry: 'Fashion',
detail: { Boss: 'Jake', Address: 'Seoul' }
}
console.dir(company, { showHidden: true, colors: false, depth: 0 });
// depth가 0이므로 detail의 value가 [Object]로 표시된다.
// 출력: { name: 'ABC corp', industry: 'Fashion', detail: [Object] }
showHidden : true일 경우 객체의 non-enumrable 열거 불가능한 값이나 symbol 값들도 출력한다. 기본값은 false이다.
colors: true일 경우 메세지 출력 시 색상 구분과 함께 출력된다.
depth: 출력 시 객체의 깊이 설정을 할 수 있다. 아래와 같은 복잡한 객체 구조를 출력할 경우에 용이하다.
let company = {
a: {
b: {
c: {
d: {
e: {
f: {
g: "Abc corp",
},
},
},
},
},
},
};
console.dir(company, {depth: 2});
// 출력: { a: { b: { c: [Object] } } }
console.table
객체를 표 형태로 출력한다.
let company = {
name: "ABC corp",
industry: "Fashion",
detail: { Boss: "Jake", Address: "Seoul" },
};
console.table(company);
// 출력:
┌──────────┬────────┬─────────┬────────────┐
│ (index) │ Boss │ Address │ Values │
├──────────┼────────┼─────────┼────────────┤
│ name │ │ │ 'ABC corp' │
│ industry │ │ │ 'Fashion' │
│ detail │ 'Jake' │ 'Seoul' │ │
└──────────┴────────┴─────────┴────────────┘
console.time & console.timeEnd
시간을 잴때 사용한다.
console.time("run");
for (let i = 0; i < 60; i++) {
i++;
}
console.timeEnd("run");
// 출력 : "run: 0.138ms"
time과 timeEnd사이의 시간을 재서 출력해준다. 위의 예시는 for문이 동작한 시간을 재어 출력한다.
console.trace
해당 코드의 호출 위치를 출력해준다. 어느 경로로 함수가 불러졌는지 라인 넘버와 파일 위치 등이 출력된다.
function hello() {
hi();
}
function hi() {
console.log("hi");
console.trace();
}
hello();
// 출력:
hi
Trace
at hi (C:\Users\OneDrive\Project\node\console\main.js:60:11)
at hello (C:\Users\OneDrive\Project\node\console\main.js:55:3)
at Object.<anonymous> (C:\Users\OneDrive\Project\node\console\main.js:63:1)
at Module._compile (node:internal/modules/cjs/loader:1101:14)
at Object.Module._extensions..js (node:internal/modules/cjs/loader:1153:10)
at Module.load (node:internal/modules/cjs/loader:981:32)
at Function.Module._load (node:internal/modules/cjs/loader:822:12)
at Function.executeUserEntryPoint [as runMain] (node:internal/modules/run_main:81:12)
at node:internal/main/run_main_module:17:47
위의 예제는 hi라는 함수 호출 위치를 출력한다.
console.count & console.countReset
함수가 몇번 호출되었는지 출력한다.
function call() {
console.count("paradise");
}
call();
call();
// 출력:
paradise: 1
paradise: 2
function call() {
console.count("paradise");
}
call();
console.countReset("paradise");
call();
call();
// 출력:
paradise: 1
paradise: 1
paradise: 2
countReset은 count를 초기화시키는 기능을 한다.
공감❤은 큰 힘이 됩니다. 😉
'백엔드 > Node.js' 카테고리의 다른 글
zsh: command not found: nodemon 해결하기 (0) | 2024.03.17 |
---|---|
[Node.js] Buffer란? / Buffer.alloc, Buffer.allocUnsafe, Buffer.from (0) | 2023.03.13 |
[Node.js] Node.js에서 파일명 변경하기 / fs.rename / fsPromises.rename / fs.renameSync (0) | 2023.03.07 |
[Node.js] Global object - __dirname, __filename, exports, module, require (0) | 2022.08.22 |