일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- JavaScript
- next.js
- MySQL
- 프로그래머스 신규아이디추천
- 프로그래머스
- 티스토리챌린지
- 좌표거리구하기
- 키패드누르기풀이
- 정렬 알고리즘
- 프로그래머스 자바스크립트
- 프로그래머스 자바스크립트 풀이
- binary search
- Javascript 정렬
- 자바스크립트 배열
- TypeScript
- mysql스키마
- 타입스크립트
- 오블완
- 자바스크립트 정렬
- 알고리즘
- 정규표현식문제
- 자료구조
- 맨해튼거리
- 깃허브
- node.js
- 맨해튼거리예제
- js 알고리즘
- TS
- Javascript sort
- 자바스크립트 알고리즘
- Today
- Total
FE PARADISE
[Node.js] Global object - __dirname, __filename, exports, module, require 본문
[Node.js] Global object - __dirname, __filename, exports, module, require
PARADISE247 2022. 8. 22. 23:59Node.js Global object 에 대하여
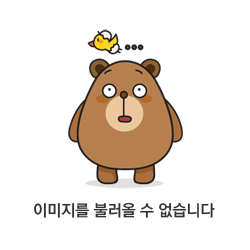
module
module은 하나의 파일 혹은 여러 파일이나 폴더가 될 수도 있다.
core modules
node.js에 내장되어 있는 모듈이다. require을 이용해서 쉽게 불러와 사용할 수 있다.
const http = require('http'); // http 서버를 구축
const fs = require('fs'); // 파일 시스템
const assert = require('assert'); // 테스트에 용이한 assertion 기능 포함
const path = require('path'); // 파일 경로와 관련된 기능
const process = require('process'); // 최근 node.js의 프로세스에 대한 정보와 제어기능
const querystring = require('node:querystring'); // url parsing, formatting 기능
const url = require('url'); // url 정보를 문자로 변환 혹은 parsing 하는 기능
local modules
개발자가 node.js 안에서 만들어내는 module이다.
// example
exports.sum = function(a,b) {
return a+b;
}
third party modules
Node Package Manager(NPM)에 의해서 사용가능한 모듈이다. 개발자가 자신의 프로젝트에 설치하거나 전역으로 설치하는 모듈들을 뜻한다. 예시로 express, angular, react 등이 있다. ( => npm install 로 설치하는 모듈들 )
__dirname & __filename
__dirname
실행중인 모듈의 디렉토리 이름을 출력한다.
___filename
실행중인 모듈의 파일 이름을 출력한다.
// app.js
console.log(__dirname);
// -> /Users/hifen/Desktop/Project/node/app
console.log(__filename);
// -> /Users/hifen/Desktop/Project/node/9-path/app.js
exports
module.exports 와 같은 기능을 한다. 공식 문서에는 module.exports의 짧은 버전이라고 소개되어 있다.
다른 모듈로 해당 모듈을 내보내기 위해서 사용한다.
module.exports와 exports 둘다 module.exports를 return한다.
console.log(module.exports === exports); // true
exports = {}; // exports에 새로운 object를 할당
console.log(module.exports === exports); // false
처음에는 exports는 module.exports를 참조하는 참조값을 가진다. 하지만 위와 같이 새로운 object 즉 새로운 값을 할당하면
exports 는 module.exports 다른 객체가 된다.
require
require(id)
- id : module의 이름이나 경로
- return: export된 모듈의 내용을 return 한다.
// calc.js
function sum(a,b) {
return a+b;
}
module.exports.sum = sum;
위와 같이 calc.js에서 sum이라는 함수를 만들어 module로 export 했다.
// app.js
const add = require('./calc.js');
console.log(add.sum(1,2)); // 3
app.js에서 calc.js의 sum 함수를 사용하고자 calc.js 모듈을 require을 통해 불러온다.
불러온 module을 add에 할당했다.
그 결과 add.sum 을 이용해 calc 모듈의 sum 함수를 사용할 수 있다.
참고 자료
https://www.geeksforgeeks.org/node-js-modules/
Node.js Modules - GeeksforGeeks
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
www.geeksforgeeks.org
https://nodejs.org/dist/latest-v18.x/docs/api/globals.html
Global objects | Node.js v18.7.0 Documentation
Global objects# These objects are available in all modules. The following variables may appear to be global but are not. They exist only in the scope of modules, see the module system documentation: The objects listed here are specific to Node.js. There ar
nodejs.org
'백엔드 > Node.js' 카테고리의 다른 글
zsh: command not found: nodemon 해결하기 (1) | 2024.03.17 |
---|---|
[Node.js] Buffer란? / Buffer.alloc, Buffer.allocUnsafe, Buffer.from (0) | 2023.03.13 |
[Node.js] Node.js에서 파일명 변경하기 / fs.rename / fsPromises.rename / fs.renameSync (0) | 2023.03.07 |
[Node.js / Javascript] console에 대하여 - console 종류 (0) | 2023.02.21 |